[Java 杂记]Arrays.asList方法遇到的坑
1.Arrays.asList使用限制
Arrays.asList是将数组转换成集合的方法
1.该方法适用于对象型数据的数组(String、Integer…)
1 2 3 4 5 6 7 8 9 10
| public static void main(String[] args) { List<String> strings = Arrays.asList("a", "b", "c"); char[] chars = "abc".toCharArray();
List<char[]> chars1 = Arrays.asList(chars); }
|
Java基本类型(byte/short/int/long/float/double/char/boolean)的数组(byte[]/short[]/int[]/long[]/float[]/double[]/char[]/boolean[])不能直接通过Arrays.asList方法转换为List,因为List的元素必须是包装类。在Java8之前,想要实现这种转换只能通过循环。对于int, long, double三种基本类型,Java8提供的新特性Stream则可以让我们在一行之内解决这个问题
1 2 3 4
| int [] ints = {1,2,3}; List<Integer> integers = Arrays.stream(ints).boxed().collect(Collectors.toList());
Arrays.stream(ints).mapToObj(value -> (Integer)value).collect(Collectors.toList());
|
那char这种的基本类型只能通过循环来转换成集合吗?
1 2 3 4 5 6
| char[] chars = "abc".toCharArray(); List list = new ArrayList(); for (char aChar : chars) { list.add(aChar); } list.forEach(o -> System.out.print(o + " "));
|
解决方法:1.通过第三方api,例如Guava
jar 包提供的 Chars.asList(
)方法 2.使用JDK 8 提供的 lambda
流式编程
1 2 3 4 5 6
| List<Character> characters = Chars.asList(chars); characters.forEach(o -> System.out.print(o + " "));
List<Character> characterList = new String(chars).chars().mapToObj(value -> (char) value).collect(Collectors.toList()); characterList.forEach(o -> System.out.print(o + " "));
|
2.返回的集合是只读的,不支持add()、remove()、clear()等方法
1 2
| List<String> strings = Arrays.asList("a", "b", "c");//使用包装对象String strings.add("d");//报异常java.lang.UnsupportedOperationException
|
Arrays.asList返回ArrayList不是java.util包下的,而是java.util.Arrays.ArrayList
它是Arrays类自己定义的一个静态内部类,这个内部类没有实现add()、remove()方法,而是直接使用它的父类AbstractList的相应方法。
而AbstractList中的add()和remove()是直接抛出java.lang.UnsupportedOperationException异常的!
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
|
public void add(int index, E element) { throw new UnsupportedOperationException(); }
public E remove(int index) { throw new UnsupportedOperationException(); }
|
可以通过下面的方法解决
1 2 3
| List<String> strings = Arrays.asList("a", "b", "c"); ArrayList<String> stringArrayList = new ArrayList<>(strings); stringArrayList.add("d");
|
2.Java——日期格式化YYYYMMdd与yyyyMMdd的区别

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| public static void main(String[] args) { //YYYY 是表示:当天所在的周属于的年份,一周从周日开始,周六结束,只要本周跨年,那么这周就算入下一年。 //2019-12-29至2020-1-4跨年周 Calendar calendar = Calendar.getInstance(); //2019-12-28 calendar.set(2019, Calendar.DECEMBER, 28); Date strDate1 = calendar.getTime(); //2019-12-29 calendar.set(2019, Calendar.DECEMBER, 29); Date strDate2 = calendar.getTime(); // 2019-12-31 calendar.set(2019, Calendar.DECEMBER, 31); Date strDate3 = calendar.getTime(); // 2020-01-01 calendar.set(2020, Calendar.JANUARY, 1); Date strDate4 = calendar.getTime(); DateFormat df1 = new SimpleDateFormat("yyyyMMdd"); DateFormat df2 = new SimpleDateFormat("YYYYMMdd"); //yyyyMMdd System.out.println("yyyyMMdd"); System.out.println("2019-12-28: " + df1.format(strDate1)); System.out.println("2019-12-29: " + df1.format(strDate2)); System.out.println("2019-12-31: " + df1.format(strDate3)); System.out.println("2020-01-01: " + df1.format(strDate4)); //YYYYMMdd System.out.println("YYYYMMdd"); System.out.println("2019-12-28: " + df2.format(strDate1)); System.out.println("2019-12-29: " + df2.format(strDate2)); System.out.println("2019-12-31: " + df2.format(strDate3)); System.out.println("2020-01-01: " + df2.format(strDate4)); }
|

结果:
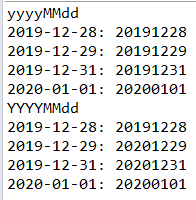