7大设计原则和23种设计模式
1.前言
- 设计模式不是一种新的语言,也不是什么新api,更不是什么新语法。
- 设计模式是前辈们不断总结,不断打磨出的设计方法。不同的设计模式适用于不同的场景。
- 设计模式公认的有23种,分别对应23种不同的设计场景。
- 千万不要认为任何一种设计模式能解决任何问题,每一种设计模式只能用于适用的场景,不是万能的。
- 设计模式不是万能的,有优点也有缺点,不能为了适用设计模式而使用设计模式。切记防止‘’‘模式的滥用’
- 23种设计模式,背后其实是7大设计原则。也就是说,每一个设计模式都归属于一个或者多个设计原则
- 七大设计原则背后总结为一个字:分
- 7大设计原则分别是:
- 单一职责原则
- 接口隔离原则
- 里氏替换原则
- 迪米特法则(最少知道原则)
- 依赖倒置原则
- 开闭原则(OCP)
- 组合优于继承原则
1.单一职责原则
每个方法,每个类,每个框架都只负责一件事情
例如:Math.round()只负责完成四舍五入方法,其它不管(方法)
Read类只负责文件的读取(类)
SpringMVC只负责简化MVC的开发(框架)
反例:
1 | /** |
正例:
1 | /** |
2.开闭原则(OCP)
1.对扩展新功能开放
2.对修改旧功能关闭
反例:
1 | /** |
正例:
1 | /** |
3.接口隔离原则
1.客户端不应该依赖它不需要的接口
2.类间的依赖关系应该建立在最小接口上
反例:
1 | /** |
正例:
1 | /** |
4.依赖倒置原则
上层不应该依赖于下层,上层和下层都应该依赖于抽象的接口
反例:
1 | /** |
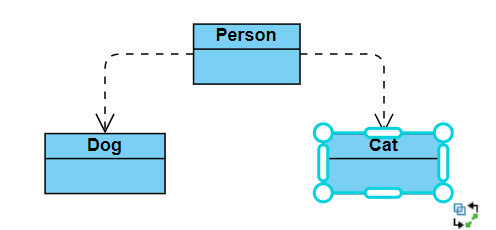
正例:
1 | /** |
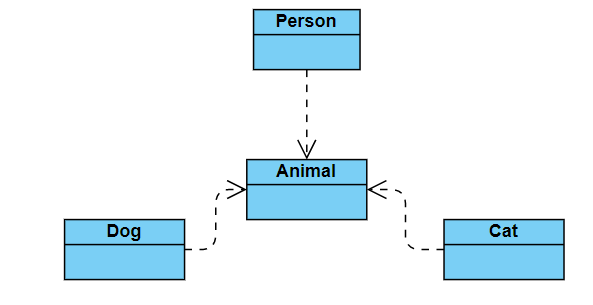
5.迪米特法则(最少知道原则)
迪米特法则,也叫最少知道原则(封装)
一个类,对于其他类,要知道的越少越好
只和朋友通信,什么是朋友:1、类中的字段2、方法的返回值 3、方法的参数 4、方法中实例化出的对象
反例:
1 | /** |
反例:
1 | /** |
6.里氏替换原则
任何能使用父类对象的地方,都应该能透明地替换为子类对象。子类对象替换父类对象,语法不会报错,业务逻辑也不会出问题
反例:
1 | /** |
7.组合优于继承
类与类之间的关系有3种:
1.继承:子类继承父类
2.依赖: 一个类的对象作为另一个类的局部变量
3.关联:一个类的对象作为另一个类的字段(属性)
“关联”可以细分为:1.组合(关系强,鸟和翅膀) 2.聚合(关系弱,大雁和雁群)
组合优于继承中的组合没有分的那么细,其实指的的就是关联关系。
例A:
1 | /** |
例B:
1 | /** |
例C:
1 | /** |
例D:
1 | /** |
例E:
1 | /** |
23种设计模式
1.简单工厂模式
反例:
1 | /** |
正例:
1 | /** |
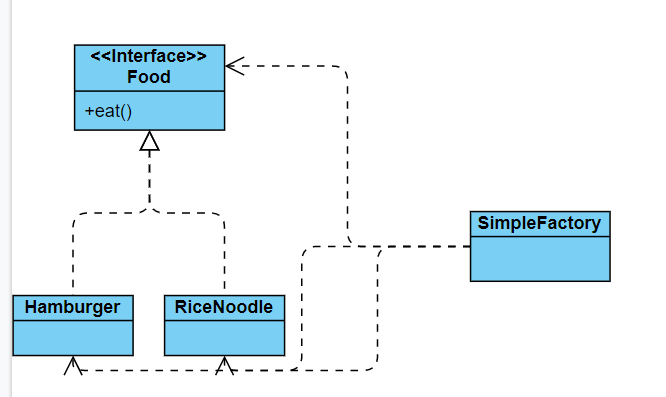
2.工厂方法模式
1 | /** |
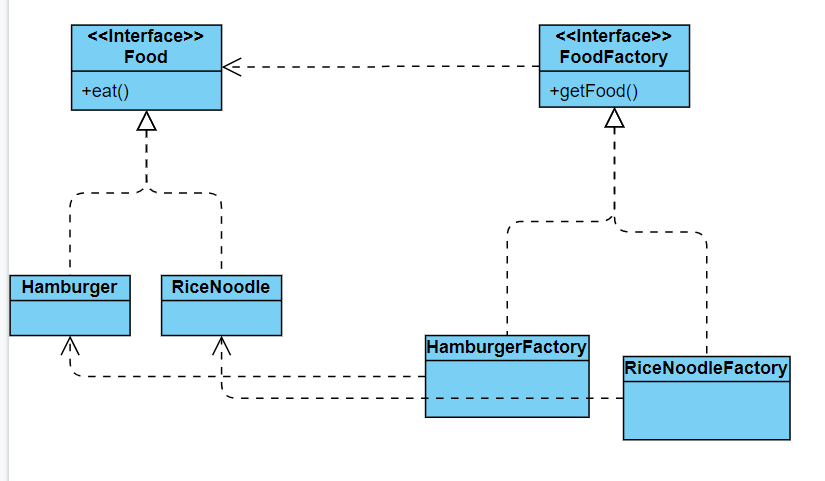
3.抽象工厂
1 | /** |
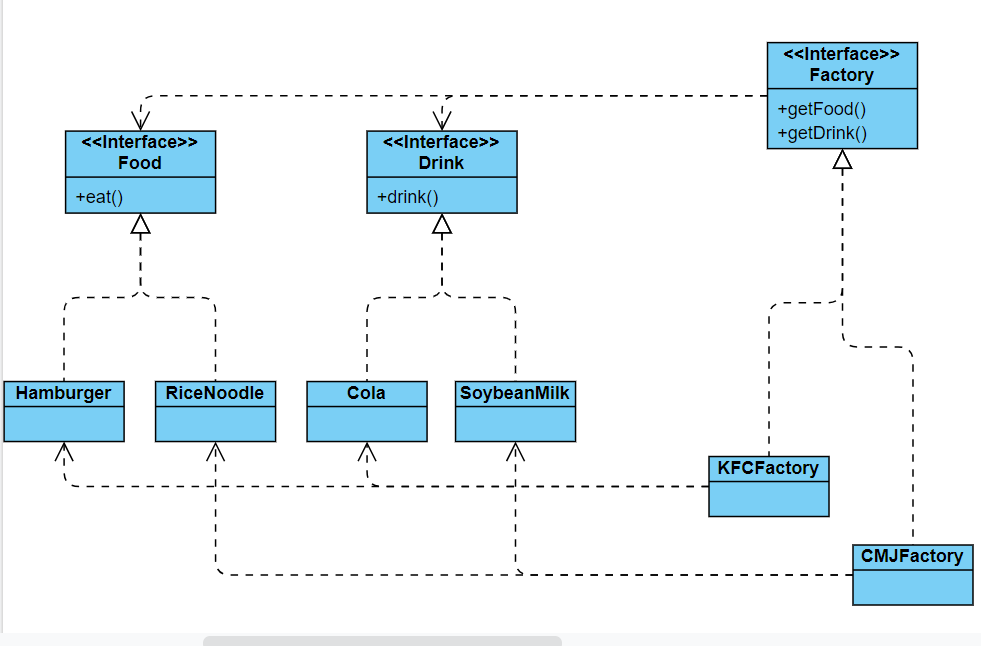
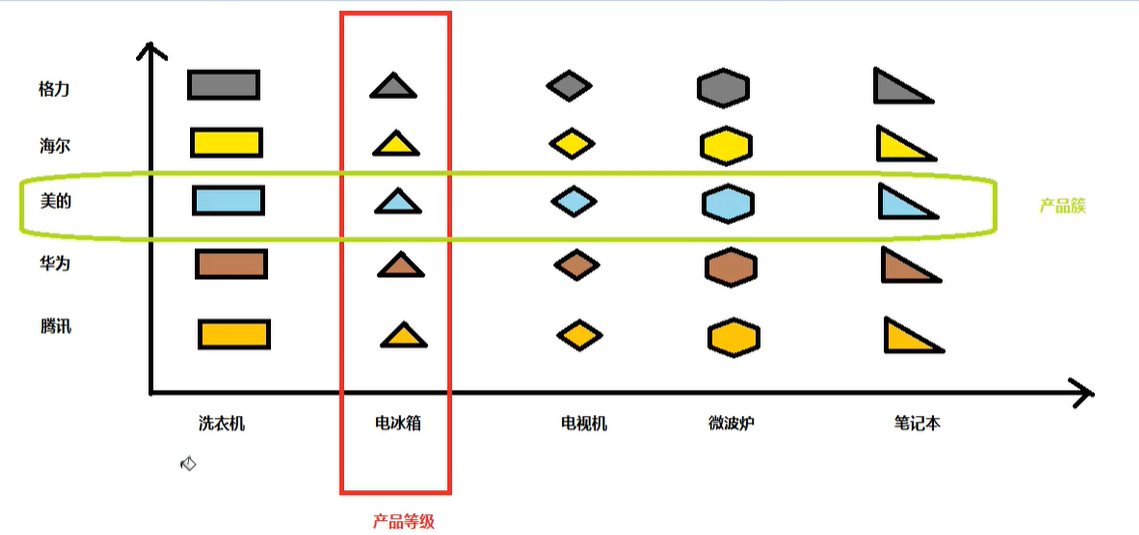
4.代理模式
例A:
1 | /** |
例B:
1 | /** |
例C:
1 | /** |
例D:
1 | /** |
1 | /** |
5.建造者模式
1 | /** |
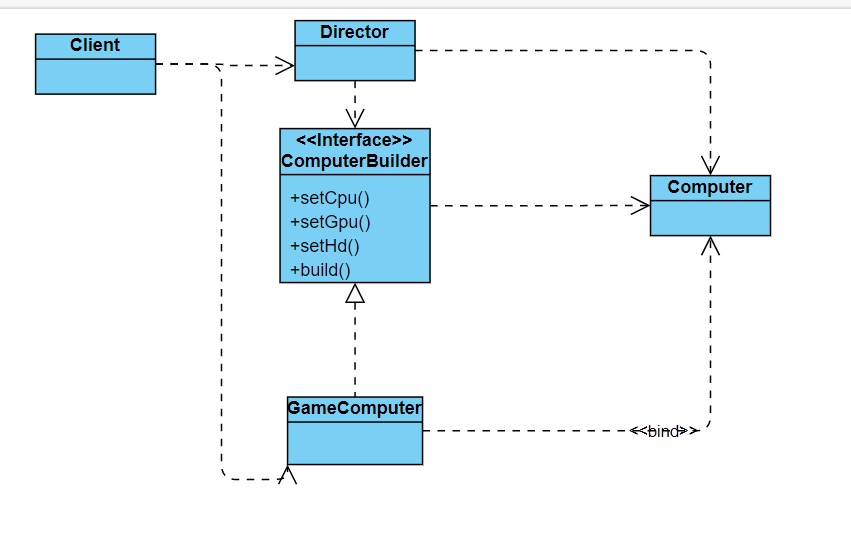